Reporting & Reconciliation
Reporting APIs
Aeropay offers a number of APIs that can be used to fetch all transactions, users, and aggregated transaction totals for your merchant. Your team can leverage these endpoints for reporting and reconciliation of funds or analytics on transaction and user activity.
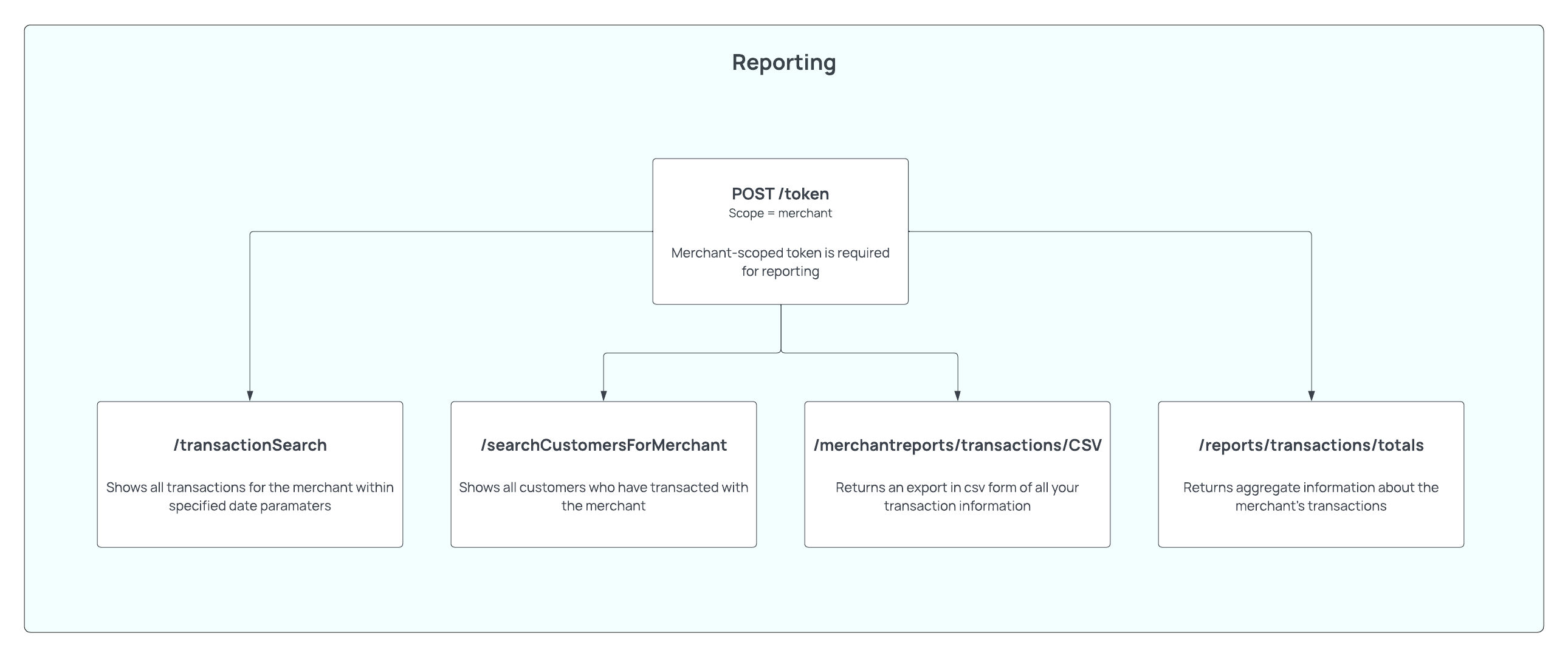
1. POST /transactionSearch
Searching for a transaction can be done using the GET /transactionSearch
endpoint. Transactions can be searched by merchant, date, user information, transaction uuid, or transaction id. Transaction search API will return a list of transactions for the specified search.
HTTP request
Sandbox - POST https://staging-api.aeropay.com/transactionSearch
Production - POST https://api.aeropay.com/transactionSearch
Request parameters
Parameter | Required? | Type | Description |
---|---|---|---|
merchantId | Yes | String | MerchantId of the transactions being searched |
search | Yes | String | The value being searched for, based on the searchType |
searchType | Yes | String | The transaction attribute being searched on: transactionId, uuid, userId, phone, email, name, locationId, merchantId, default. searchType default will fetch all transactions for your merchant |
dateStart | No | String | Start date of search range in ISO 8601 format %m-%d-%Y %H:%M:%S |
dateEnd | No | String | End date of search range in ISO 8601 format %m-%d-%Y %H:%M:%S |
timezone | No | String | Timezone of date range. Ex: America/Chicago |
page | No | String | Page number. |
perPage | No | String | Number of transactions returned per page. Default to 50. |
orderBy | No | String | Order search by 'asc' or 'desc' |
sortBy | No | String | Sort search by value: id, startTime, amount, userId. Defaults to userId. |
Code Example - Request
curl --request POST \
--url https://staging-api.aeropay.com/transactionSearch \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: token' \
--data '
{
"searchType": "userId",
"merchantId": "123",
"search": "543",
"dateStart": "05-13-1995 00:00:00",
"dateEnd": "04-20-2023 23:59:59",
"timezone": "America/Chicago"
}
'
Code Example - Response
{
"data": {
"id": "1331210",
"status": "pending",
"userId": "123543",
"merchantId": "575",
"locationId": "675",
"title": "Online Transaction",
"amount": "174.00",
"apFee": "3.12",
"createdDate": "1686864379",
"modifiedDate": "1686864379",
"transactionId": "None",
"handshakeType": "ol",
"duration": "00:00:00",
"deviceId": "None",
"paymentType": "payment",
"attributes": [],
"userAccountId": "1",
"merchantAccountId": "392",
"internalAmount": "17400",
"uuid": "76c2c9e0-c756-4ccd-b980-2f72d15e3dc0"
}
}
2. GET /searchCustomersForMerchant
All customers who have transacted with the merchant can be accessed via our GET /searchCustomersForMerchant
API.
HTTP request
Sandbox - GET https://staging-api.aeropay.com/searchCustomersForMerchant
Production - GET https://api.aeropay.com/searchCustomersForMerchant
Query parameters
Parameter | Required? | Type | Description |
---|---|---|---|
merchantId | Yes | String | MerchantId of the transactions being searched |
page | No | String | Page number. |
perPage | No | String | Number of transactions returned per page. Default to 50. |
orderBy | No | String | Order search by 'asc' or 'desc' |
sortBy | No | String | Sort search by value: id, startTime, amount, userId. Defaults to userId. |
Code Example - Request
curl --request GET \
--url 'https://staging-api.aeropay.com/searchCustomersForMerchant?merchantId=234&perPage=2&sortBy=id&orderBy=asc' \
--header 'accept: application/json'
--header 'authorizationToken: Bearer {{token}}'
Code Example - Response
{
"customers": [
{
"userId": "13000",
"firstName": "Harry",
"lastName": "Cohen",
"email": "harrycohen@gmail.com",
"phone": "+13334442345",
"hasValidated": "None",
"customerId": "None",
"receivesEmail": "0",
"totalTransactionAmount": "200",
"totalTransactionCount": "1",
"firstTransaction": "2023-08-23 20:54:16",
"lastTransaction": "2023-08-23 20:54:16",
"currency": "USD",
"profImgUrl": null
},
{
"userId": "12999",
"firstName": "Luke",
"lastName": "Doe",
"email": "lucasdoe@gmail.com",
"phone": "+144423287978",
"hasValidated": "None",
"customerId": "None",
"receivesEmail": "0",
"totalTransactionAmount": "3765",
"totalTransactionCount": "3",
"firstTransaction": "2023-08-22 19:08:40",
"lastTransaction": "2023-08-23 18:56:43",
"currency": "USD",
"profImgUrl": null
}
],
"paging": {
"totalPages": 4,
"totalItems": 193,
"currentPage": 1,
"itemsPerPage": 2
},
"success": true
}
3. GET /reports/transactions/totals
The GET /reports/transactions/totals
endpoint returns aggregated information about the merchant’s transactions over a given time period.
HTTP request
Sandbox - GET https://staging-api.aeropay.com/reports/transactions/totals
Production - GET https://api.aeropay.com/reports/transactions/totals
Query parameters
Parameter | Required? | Type | Description |
---|---|---|---|
merchantId | Yes | String | MerchantId of the transactions in report. |
dateBegin | Yes | String | Start date of report range in DD-MM-YYYY format. |
dateEnd | Yes | String | End date of report range in DD-MM-YYYY format. |
timezone | Yes | String | ISO 8601 timezone value. Default is UTC. |
Code Example - Request
curl --request GET \
--url 'https://staging-api.aeropay.com/reports/transactions/totals?dateBegin=05-20-2020&dateEnd=07-23-2023&timezone=America%2FNew_York' \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}'
Code Example - Response
{
"chargeTotal": "140,509.90",
"tipTotal": "0.00",
"feeTotal": "4,344.19",
"rewardsTotal": "0.00",
"payoutsTotal": "0.00",
"refundsTotal": "0.00",
"netTotal": "136,165.71"
}
4. GET /reports/transactions/CSV
The GET /merchantreports/transactions/CSV
endpoint will return a csv export of all your transaction information. The CSV will be sent to the emails specified.
HTTP request
Sandbox - GET https://staging-api.aeropay.com/reports/transactions/CSV
Production - GET https://api.aeropay.com/reports/transactions/CSV
Query parameters
Parameter | Required? | Type | Description |
---|---|---|---|
merchantId | Yes | int32 | MerchantId of the transactions in report. |
dateBegin | Yes | String | Start date of report range in DD-MM-YYYY format. |
dateEnd | Yes | String | End date of report range in DD-MM-YYYY format. |
timezone | Yes | String | ISO 8601 timezone value. Default is UTC. |
page | No | int32 | If specified along with size, set page number. |
size | No | int32 | If specified along with page, set page size. |
No | string | Comma separated list of emails to receive the CSV report. |
Code Example - Request
curl --request GET \
--url 'https://staging-api.aeropay.com/reports/transactions/CSV?merchantId=1234&dateBegin=04-20-2020&dateEnd=04-21-2020&timezone=America%2FNew_York' \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}'
Updated 5 months ago