Manage Transactions, Users, or Banks
Managing Transactions
Option 1: Aeropay Merchant Portal
For the lowest lift implementation, all payments can be managed in our Aeropay merchant portal. From here, employees can void, refund, and capture transactions as well as download reports of transactions in our pre-built UI.
Option 2: API Integration
Alternatively, you can fully integrate with Aeropay's transaction management APIs to allow your employees to manage payments in your own back office.
Tokens for Managing Transactions
All API endpoints for managing transactions require a merchant-scoped token. All tokens have a time to live (TTL) of 30 minutes.
curl --request POST \
--url https://api.sandbox-pay.aero.inc/token \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--data '{"scope":"merchant"}'
Regular Transactions
Retrieve information about an individual transaction using the GET /transaction
endpoint. Transactions can be fetched either by the Aeropay transaction ID or the transaction UUID sent from your system.
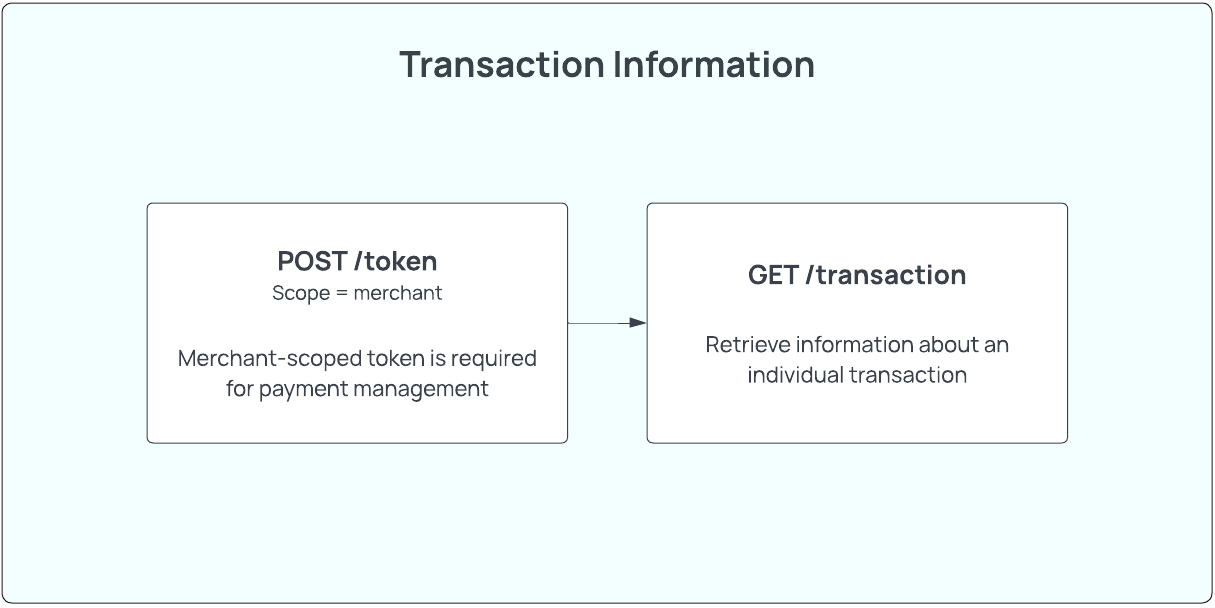
GET /transaction
curl --request GET \
--url 'https://api.sandbox-pay.aero.inc/transaction?transactionId=123' \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}'
Preauthorized Transactions
Preauthorized transactions do not initiate the movement of any funds, but instead store the details of a transaction that must be captured later by an employee. Because preauthorized transactions are not processed until captured, these types of transactions can be updated with PATCH /preauthTransaction
or deleted with DELETE /preauthTransaction
. Preauthorized transactions can be captured with POST /capturePreauthTransaction
endpoint.
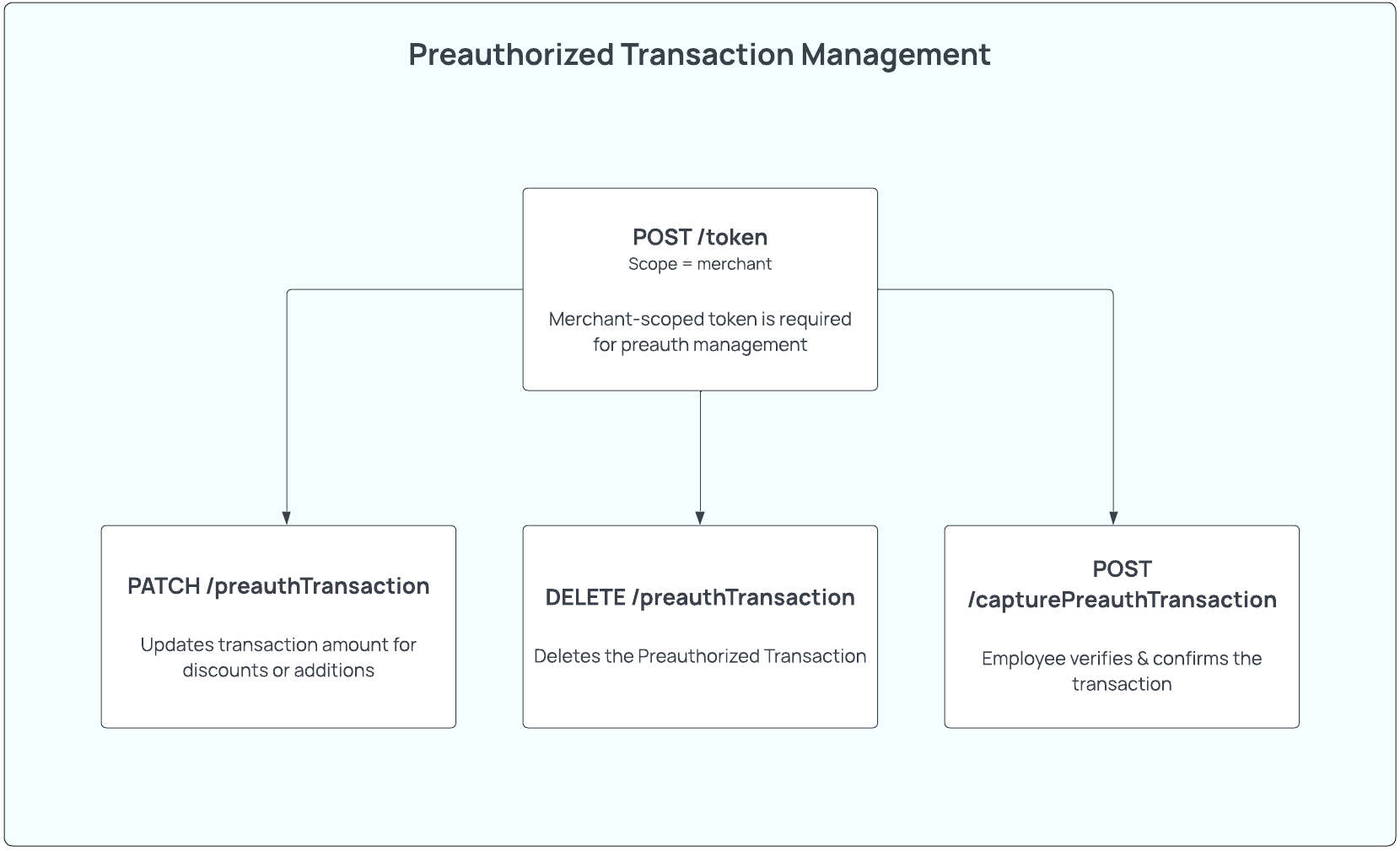
PATCH /preauthTransaction
Preauthorized transactions are updated using the PATCH /preauthTransaction
endpoint. The total amount and tip amount can be increased or decreased.
curl --request PATCH \
--url https://api.sandbox-pay.aero.inc/preauthTransaction \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"id": "1234",
"amount": "9.00"
}
'
DELETE /preauthTransaction
Preauthorized transactions can be deleted using the DELETE /preauthTransaction
endpoint. If a preauthorized transaction has already been captured, it cannot be deleted and instead need to be refunded via GET /reverseTransaction
.
curl --request DELETE \
--url 'https://api.sandbox-pay.aero.inc/preauthTransaction?id=123' \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}'
POST /capturePreauthTransaction
Once an employee verifies and confirms that an order has been completed, a preauthorized transaction can be captured using the POST /capturePreauthTransaction
. This endpoint will initiate the movement of funds for the transaction.
POST /capturePreauthTransaction
will return a transactionId that can be used to track the transaction going forward.
curl --request POST \
--url https://api.sandbox-pay.aero.inc/capturePreauthTransaction \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}' \
--data '{"id":"1234"}'
GET /preauthTransaction
Retrieve information about an individual transaction using the GET /preauthTransaction
endpoint. Transactions can be fetched either by the Aeropay preauth ID or the transaction UUID sent from your system.
curl --request POST \
--url https://api.sandbox-pay.aero.inc/preauthTransaction?uuid=ed07e6a3-3493-4cb7-ac99-07ebca7b36e7 \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}'
Refunds
Transactions can be voided, refunded, or partially refunded with GET /reverseTransaction
.
If GET /reverseTransaction
is called on the day the payment was made, the transaction will be voided. If GET /reverseTransaction
is used to issue a partial refund or is called on a subsequent day, the transaction will be batched and refunded.
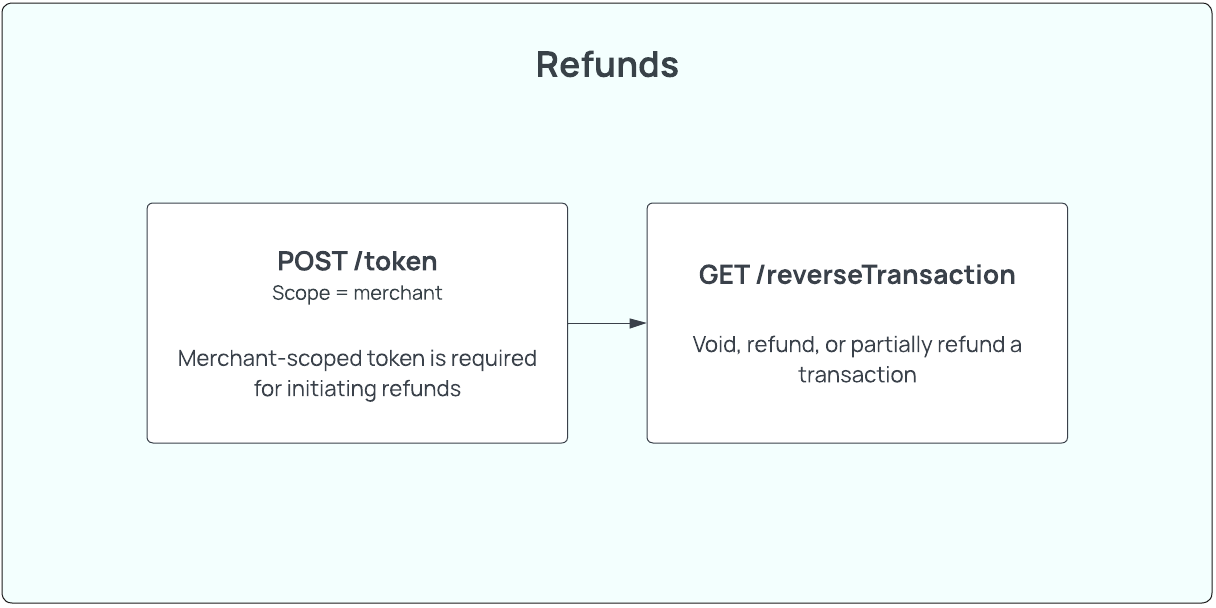
GET /reverseTransaction
curl --request GET \
--url 'https://api.sandbox-pay.aero.inc/reverseTransaction?transactionId=123&amount=5&uuid=550e8400-e29b-41d4-a716-446655440000' \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}'
If an amount is not specified as a query parameter, the full transaction amount will be refunded/voided.
There is no time limit on refunds, but we recommend setting 90 day refund limits.
Managing Bank Accounts
Aeropay recommends that you prompt users to link a bank account during registration, at the time of checkout, and in the user’s profile (if applicable), as these are universally recognized places a user would expect to update their bank. Doing so has been shown to result in higher user conversion.
A users's existing connected banks can be accessed in the bankAccounts
array in the user object returned from GET /user
. If a user has connected to more than one bank account, the bankAccounts array will return multiple available accounts. The default bank will always have the value of isSelected: 1
.
PATCH /userBankAccount
To update the users's default bank, you can invoke the PATCH /userBankAccount endpoint to update which bank account has the attribute isSelected: 1
.
curl --request PATCH \
--url https://api.sandbox-pay.aero.inc/userBankAccount \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"bankAccountId": "12567382"
}
'
DELETE /userBankAccount
To remove a bank from displaying on your platform, you can invoke the DELETE /userBankAccount
endpoint.
curl --request DELETE \
--url https://api.sandbox-pay.aero.inc/userBankAccount \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"bankAccountId": "1227942"
}
'
Managing User Information Adjustments
If users need to request changes to the information in their Aeropay profile, you can invoke the PATCH /user
API call with a merchant-scoped token.
Changes to the User's email or name can simply be made via a singular call to PATCH /user
.
Phone numbers are unique identifiers for Aeropay users. If changes need to be made to the User's phone number, we will require that you validate the new phone number via MFA before calling PATCH /user.
- If the new phone number is already linked to an existing Aeropay user, an error message will arrive requesting the user contact [email protected].
- If the new phone number is not already linked to an existing Aeropay user, the user's phone number will update. For security purposes, all previously linked bank accounts will be deleted and the user will have to re-link their bank accounts before making another transaction.
PATCH /user
Calling the PATCH /user endpoint will update the information for the specified user. Any request body parameters left blank will not change for the specified user.
curl --request PATCH \
--url https://api.sandbox-pay.aero.inc/user \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"userId": "456",
"first_name": "Janey",
"last_name": "Doe",
"email": "[email protected]",
"phone_number": "123-123-1234"
}
'
Updated 15 days ago