API Quick Start
Basic API Flow
The following flow visualizes a basic new user flow through user creation, bank connections, and transaction creation.
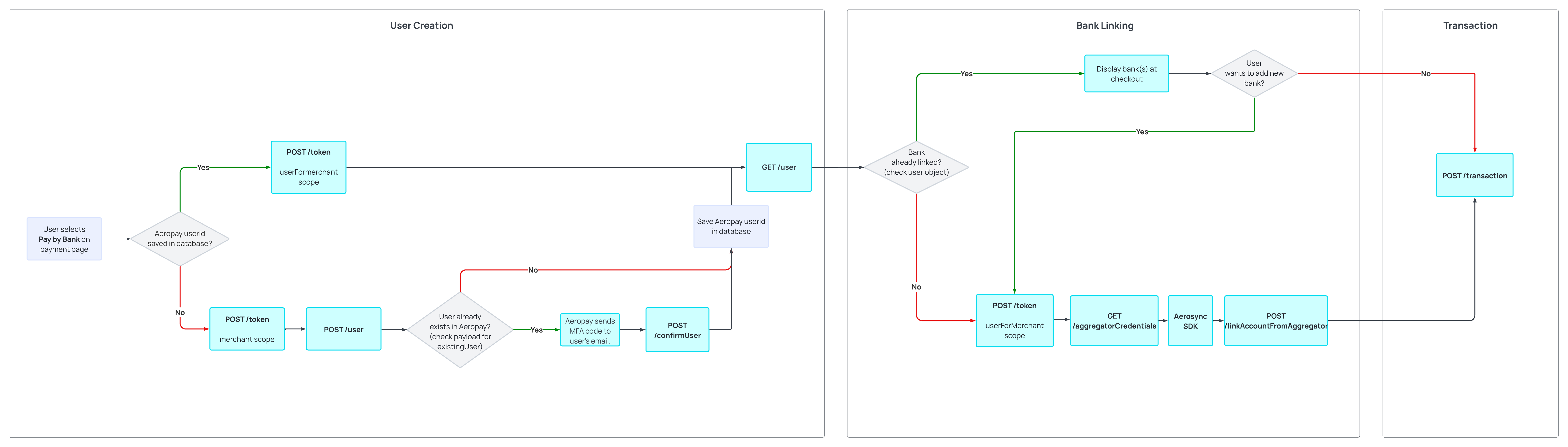
User Creation
Aeropay users are individuals, uniquely identified by a phone number, who can link bank accounts and make transactions. If you are opting for white-labeled user creation in your application, you'll need to create a user before initiating a preauthorized transaction.
An Aeropay account can be created on behalf of a user using the POST /user
endpoint. The authorizationToken used to create a user must be a merchant-scoped token.
The userId returned from this call is required to reference the user within the Aeropay system. If a user already has an account with Aeropay, their existing account information will be returned.
Bank Linking
Once a user has been created, they can go through the flow to link their bank account.
The authorizationToken used to act on behalf of a user must be a userForMerchant-scoped token.
The GET /aggregatorCredentials
endpoint will return the necessary URL to launch the aggregator widget. Launching the aggregator widget will allow the User to securely link their bank account through their bank’s website. After the aggregator widget is closed, the GET /linkAccountFromAggregator
endpoint will connect the user's bank to their Aeropay account.
Understand how to launch our aggregator widget.
Transaction
Once a user has a linked bank, they can begin to make transactions. See below some details on each transaction type, or skip right to the transaction guides: Standard Transaction Guide, Payout Transaction Guide, Preauth Transaction Guide, Subscription Transaction Guide
Option 1: Standard Transaction
To create a standard transaction, use the GET /user
endpoint to fetch user's linked bank accounts. The bankAccountId returned from this endpoint can be used to call thePOST /transaction
endpoint to specify the bank account used in the transaction.
Tipping and invoicing information can be added to transactions in the attributes of the POST /transaction
body.
Before creating the transaction, you must request a new userForMerchant-scoped token if your token has expired.
curl --request POST \
--url https://api.sandbox-pay.aero.inc/transaction \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'X-AP-Version: 2023-06-05' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"amount": "10.00",
"merchantId": "12345",
"uuid": "transaction-UUID"
}
'
Option 2: Payout Transaction
Payout transactions are payments that flow from merchant to User. These types of transactions may be used for withdrawals or rewards.
To create a payout transactions, use the GET /user
endpoint to fetch User's actively linked bank accounts. The bankAccountId returned from this endpoint can be used to call thePOST /payoutTransaction
endpoint and initiate a payout transaction.
curl --request POST \
--url https://api.sandbox-pay.aero.inc/payoutTransaction \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'X-AP-Version: 2023-06-05' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"userId": "4567",
"amount": "10.00",
"bankAccountId": "27381938"
}
'
Option 3: Preauthorized Transaction
Preauthorized transactions do not initiate the movement of any funds, but instead store the details of a transaction that must be captured later by an employee.
To create a preauthorized transactions, use the GET /user
endpoint to fetch User's actively linked bank accounts. The bankAccountId returned from this endpoint can be used to call thePOST /preauthTransaction
endpoint and create a preauthorized transaction.
Tipping and invoicing information can be added to transactions in the attributes of the POST /preauthTransaction
body.
See Manage Transactions for more detail on capturing or updating preauthorized transactions.
curl --request POST \
--url https://api.sandbox-pay.aero.inc/preauthTransaction \
--header 'Content-Type: application/json' \
--header 'accept: application/json' \
--header 'authorizationToken: Bearer {{token}}' \
--data '
{
"merchantId": "1235",
"bankAccountId": "1234567",
"amount": "10.00"
}
'
Updated 28 days ago